How to Learn .NET from Scratch: A Roadmap for Beginners
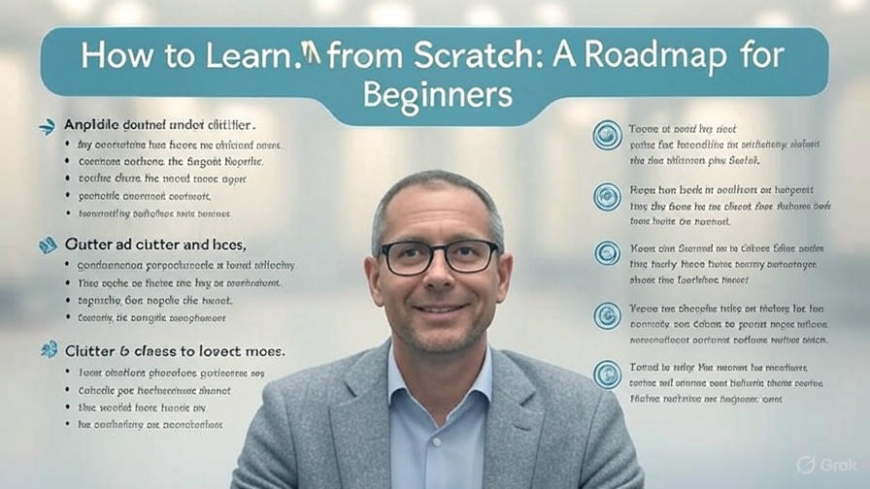
Are you looking to break into the world of software development? Learning .NET from scratch can open doors to countless career opportunities in today's tech-driven market. The .NET framework, developed by Microsoft, remains one of the most versatile and powerful platforms for building modern applications. If you're interested in pursuing a .net course online, you'll find numerous resources to help you master this technology. Additionally, obtaining a microsoft dot net certification can significantly boost your employment prospects and validate your skills to potential employers.
This comprehensive guide provides a strategic roadmap for beginners embarking on their .NET learning journey. We'll cover everything from understanding the fundamental concepts to mastering advanced techniques, helping you transform from a novice to a proficient .NET developer.
Understanding the .NET Ecosystem
Before diving into coding, it's crucial to understand what .NET actually is. The .NET framework is a software development platform developed by Microsoft that offers a controlled programming environment for building and running applications. It consists of the Common Language Runtime (CLR), which provides memory management and other system services, and an extensive class library called the Framework Class Library (FCL).
Modern .NET has evolved significantly over the years. With the introduction of .NET Core (now simply .NET), Microsoft has made the framework cross-platform, open-source, and more performant than ever before. This means you can develop .NET applications not just on Windows, but also on macOS and Linux.
Setting Up Your Development Environment for Learning .NET
To begin your journey in learning .NET from scratch, you need to set up a proper development environment. Here's what you'll need:
Installing Visual Studio
Visual Studio is the premier Integrated Development Environment (IDE) for .NET development. It comes in different editions:
-
Visual Studio Community: Free for individual developers, open-source projects, and small teams
-
Visual Studio Professional: For professional developer teams
-
Visual Studio Enterprise: For large-scale enterprise development
For beginners, Visual Studio Community is more than sufficient. When installing, make sure to select the ".NET desktop development" workload.
Alternative IDEs
If you prefer a lighter-weight option, you can use:
-
Visual Studio Code with C# extensions: A free, open-source, cross-platform code editor
-
JetBrains Rider: A powerful cross-platform .NET IDE (paid, but with a free trial)
Installing .NET SDK
The .NET Software Development Kit (SDK) includes everything you need to build and run .NET applications. Download the latest version from the Microsoft website and follow the installation instructions.
Core Programming Concepts in .NET
Now that your environment is set up, it's time to understand the core programming concepts of .NET.
C# Fundamentals
C# (pronounced "C-sharp") is the most popular programming language for .NET development. Start by learning:
-
Variables and data types
-
Operators and expressions
-
Control flow statements (if-else, switch, loops)
-
Methods and functions
-
Exception handling
Object-Oriented Programming (OOP)
.NET is built around object-oriented programming principles. Master these concepts:
-
Classes and objects
-
Inheritance and polymorphism
-
Encapsulation
-
Abstraction
-
Interfaces and abstract classes
Understanding the Common Type System
The Common Type System (CTS) in .NET ensures that objects written in different .NET languages can interact with each other. This is crucial for understanding how different parts of a .NET application work together.
Building Your First .NET Application
After establishing a solid foundation in programming concepts, it's time to create your first application. Start with something simple like a console application.
Console Applications
Console applications are text-based programs that serve as an excellent starting point for beginners. They allow you to focus on core programming concepts without the complexity of graphical interfaces.
csharp
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, .NET World!");
Console.ReadKey();
}
}
}
Windows Forms Applications
Once comfortable with console applications, move on to Windows Forms, which allows you to create desktop applications with graphical user interfaces.
ASP.NET Web Applications
ASP.NET enables you to build dynamic web applications and services. Start with a simple web page and gradually explore more complex features.
Advanced .NET Concepts
As you progress in your learning .NET from scratch journey, you'll encounter more advanced concepts that are essential for professional development.
LINQ (Language Integrated Query)
LINQ is a powerful set of features for querying data from different sources, including collections, databases, and XML documents. It makes data manipulation more intuitive and concise.
Entity Framework
Entity Framework is an Object-Relational Mapping (ORM) framework that enables developers to work with databases using .NET objects, eliminating the need for most data-access code.
Asynchronous Programming
Modern applications often need to perform operations without blocking the main thread. Asynchronous programming in .NET, using async/await keywords, allows for more responsive and efficient applications.
Web Development with ASP.NET Core
ASP.NET Core is a cross-platform, high-performance framework for building modern, cloud-based, internet-connected applications. Here's what to learn:
MVC Architecture
The Model-View-Controller pattern separates an application into three main components, making it easier to manage and scale.
Razor Pages
A simpler alternative to MVC, Razor Pages is a page-based programming model that makes building web UI easier.
Web APIs
Learn to create RESTful services that can be consumed by various clients, including web applications, mobile apps, and IoT devices.
Mobile Development with .NET
.NET allows you to build mobile applications for various platforms.
Xamarin
Xamarin enables developers to use C# to create native mobile applications for iOS and Android, sharing code across platforms.
MAUI (Multi-platform App UI)
The successor to Xamarin.Forms, MAUI allows you to build native desktop and mobile apps with a single codebase.
Database Integration in .NET
Most real-world applications require data storage and retrieval.
SQL Server Integration
Learn how to connect to and query SQL Server databases from your .NET applications.
NoSQL Database Integration
Explore integration with popular NoSQL databases like MongoDB and Azure Cosmos DB.
Cloud Integration with Azure
Microsoft Azure offers a wide range of cloud services that integrate seamlessly with .NET applications.
Azure App Service
Learn to deploy your web applications to Azure App Service for scalable and reliable hosting.
Azure Functions
Build serverless applications that automatically scale based on demand.
Testing and Debugging .NET Applications
Quality assurance is an essential part of professional software development.
Unit Testing
Learn frameworks like MSTest, NUnit, or xUnit to write automated tests for your code.
Debugging Techniques
Master the debugging tools provided by Visual Studio to find and fix issues in your applications.
Best Practices for Learning .NET Effectively
To accelerate your .NET learning journey, follow these best practices:
Consistent Practice
Dedicate regular time to coding. Even 30 minutes daily is better than several hours once a week.
Project-Based Learning
Build real projects that solve actual problems. This approach reinforces concepts and builds your portfolio.
Code Review
Have experienced developers review your code, or review open-source projects to learn industry standards.
Community Engagement
Join .NET communities, forums, and local meetups to learn from others and stay updated on the latest trends.
Career Paths in .NET Development
With .NET skills, various career paths become available:
-
Web Developer (specializing in ASP.NET)
-
Desktop Application Developer
-
Mobile App Developer (using Xamarin or MAUI)
-
Full-Stack Developer
-
Cloud Solutions Architect
-
DevOps Engineer
As your expertise grows, so do your career opportunities and earning potential. Many positions in .NET development offer competitive salaries, with senior roles commanding six-figure incomes in many markets.
Continuing Your .NET Learning Journey
Learning .NET from scratch is just the beginning. The technology landscape continually evolves, and staying current is crucial for long-term success. Keep exploring new features and best practices through Microsoft's documentation, online courses, technical blogs, and conferences. Consider contributing to open-source projects to refine your skills and build a public portfolio that showcases your abilities to potential employers.
Remember that becoming proficient in .NET development takes time and persistence. Be patient with yourself, celebrate small victories, and don't get discouraged by challenges. With dedication and the right resources, you can master .NET development and build a rewarding career in this versatile technology stack.
Frequently Asked Questions
What programming language should I learn first for .NET development?
C# is the recommended first language for anyone starting with .NET. It's specifically designed for the .NET framework, has excellent documentation, and offers a gentle learning curve while still being powerful enough for professional development.
Is Visual Studio required for .NET development?
While Visual Studio offers the most comprehensive toolset for .NET development, it's not strictly required. You can use alternatives like Visual Studio Code with appropriate extensions or JetBrains Rider. However, most professionals prefer Visual Studio for its integrated debugging, testing, and deployment features.
How long does it take to learn .NET programming?
The timeline varies based on prior programming experience, dedication, and learning pace. Complete beginners might need 3-6 months to grasp the fundamentals and another 6-12 months to become job-ready. Those with prior programming experience can progress much faster, potentially becoming productive in just a few months.
Can I build mobile apps with .NET?
Yes, you can develop cross-platform mobile applications using Xamarin or the newer .NET MAUI (Multi-platform App UI). These frameworks allow you to write code in C# and deploy to iOS, Android, and other platforms while sharing a significant portion of your codebase.
Does .NET work on platforms other than Windows?
Absolutely! With the introduction of .NET Core (now simply .NET), you can develop and run .NET applications on Windows, macOS, and various Linux distributions. This cross-platform capability has greatly expanded .NET's versatility and adoption.
What's the difference between .NET Framework and .NET Core?
The .NET Framework is the original implementation that runs only on Windows, while .NET Core (now evolved into .NET 5 and beyond) is cross-platform, open-source, and designed with modern application development in mind. Microsoft is focusing future development on .NET, making it the recommended choice for new projects.
Are there good job opportunities for .NET developers?
Yes, .NET developers are in high demand across many industries. The framework's enterprise adoption, particularly in finance, healthcare, and government sectors, ensures a steady stream of job opportunities with competitive compensation.
Can I use .NET for game development?
While not as common as engines like Unity (which actually uses C#), you can use .NET for game development. The MonoGame framework, a reimplementation of XNA, allows you to create cross-platform games with C# and .NET.
Is .NET suitable for startups or just enterprise companies?
While .NET has a strong presence in enterprise environments, it's also well-suited for startups. The modern .NET ecosystem offers lightweight, cross-platform tools that enable rapid development and scaling, making it viable for companies of all sizes.
Do I need to learn JavaScript if I'm focusing on .NET development?
For web development with ASP.NET, understanding JavaScript is beneficial as it handles client-side interactions. However, you can start with server-side .NET development first and gradually incorporate JavaScript as needed. Technologies like Blazor are also reducing the need for JavaScript in some .NET web applications.